Galaxie Blog Version 3 is launched and is now in Final Release Candidate Status
Apr 15 |
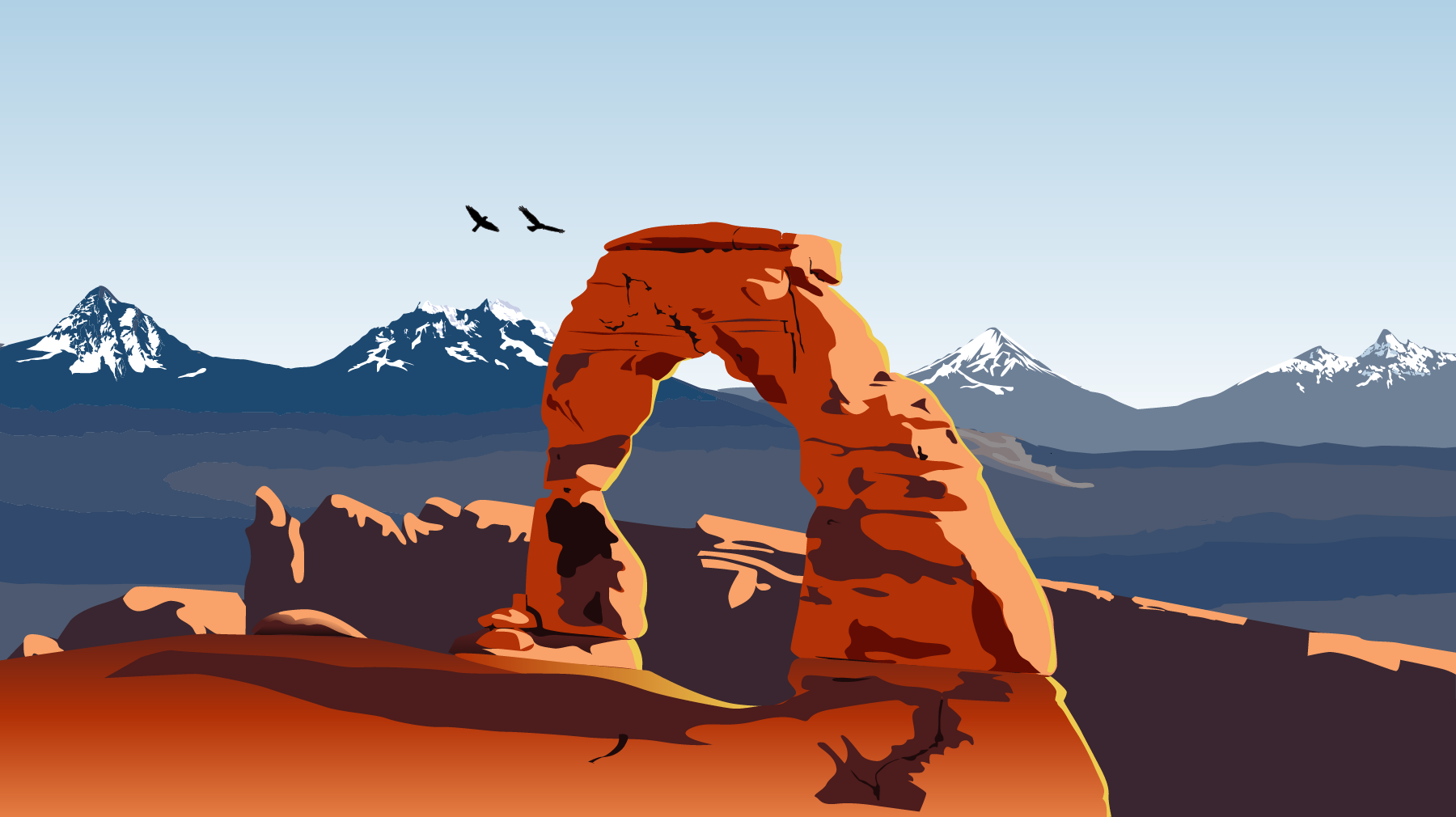
I am proud to announce that Galaxie Blog 3 is finally released. While this blog does not yet have full-fledged content management tools or e-commerce capabilities, it is intended to meet or exceed all major blog platforms' out-of-the-box core blogging functionality, such as WordPress and Wix. Unlike some other major blogging platforms, you own your data with Galaxie Blog, and Galaxie Blog only uses non-proprietary open-source libraries and is completely free.
Galaxie Blog was developed with a mobile-first priority. We have emphasized perfecting your mobile experience so you can manage the blog and create a stunning blog post with just your phone. Galaxie Blog 3.0 is also an HTML5 web application. HTML5 is a modern web standard that supports the latest multimedia features and enhances user interaction.
Galaxie Blog has extensive media support and will take the media you upload and create new media formatted for major social media platforms like Facebook and Twitter. You may also edit the images you upload to the browser.
Galaxie Blog has scores of WYSIWYG editors. You don't need to know any HTML to create a beautiful blog post. These interfaces allow you to upload images, videos, and image galleries and create maps and map routes. Galaxie Blog is perfect for the travel blogger—it offers comprehensive tools to generate and share various types of maps free of charge.
The blog also allows users to interact and share your posts on social media. It offers options to use other commenting systems, such as Disqus.
Galaxie Blog is eminently themeable. The blog has nearly 30 pre-built themes; you can develop your theme within a few minutes. Unique fonts can be applied to any theme. All of your post content will take the characteristics of your selected theme. For example, the blog sends branded emails to your subscriber base, which will be branded according to your theme.
Galaxie Blog supports more databases than any other major blog software and can be used with any modern database. You don't need an isolated database; you can keep your current database system. Galaxie Blog will also automatically create the database objects and schemas as required. It also has an automatic installer, allowing you to import data from a prior version of Galaxie Blog or other blogs, such as BlogCfc.
Galaxie Blog has extensive SEO features to improve your ranking with search engines. It also automatically structures your blog data for search engines using the most modern version of JSON-LD. Galaxie Blog also automatically generates RSS, allowing other applications to access your blog posts and create real-time feeds.
Galaxie Blog supports multiple users and user capabilities. There are nine different user roles and dozens of unique capabilities. You can create new custom roles with unique capabilities. For example, you may assign one user to moderate the blog comments and another to edit the contents of a post.
Galaxie Blog has many unique features not found in other blogs. For example, the blog allows you to use JavaScript and other advanced features in a blog post. The blog can also include a logical programming template using ColdFusion in your blog posts. The blog is also perfect for developers; it can display code and be tailored to support the display of hundreds of different programming languages.
There are many more Galaxie Blog features that I will blog about in the future.
Happy Blogging!
Related Entries
Tags
Galaxie Blog 3.0This entry was posted on April 15, 2022 at 1:56 PM and has received 1035 views.